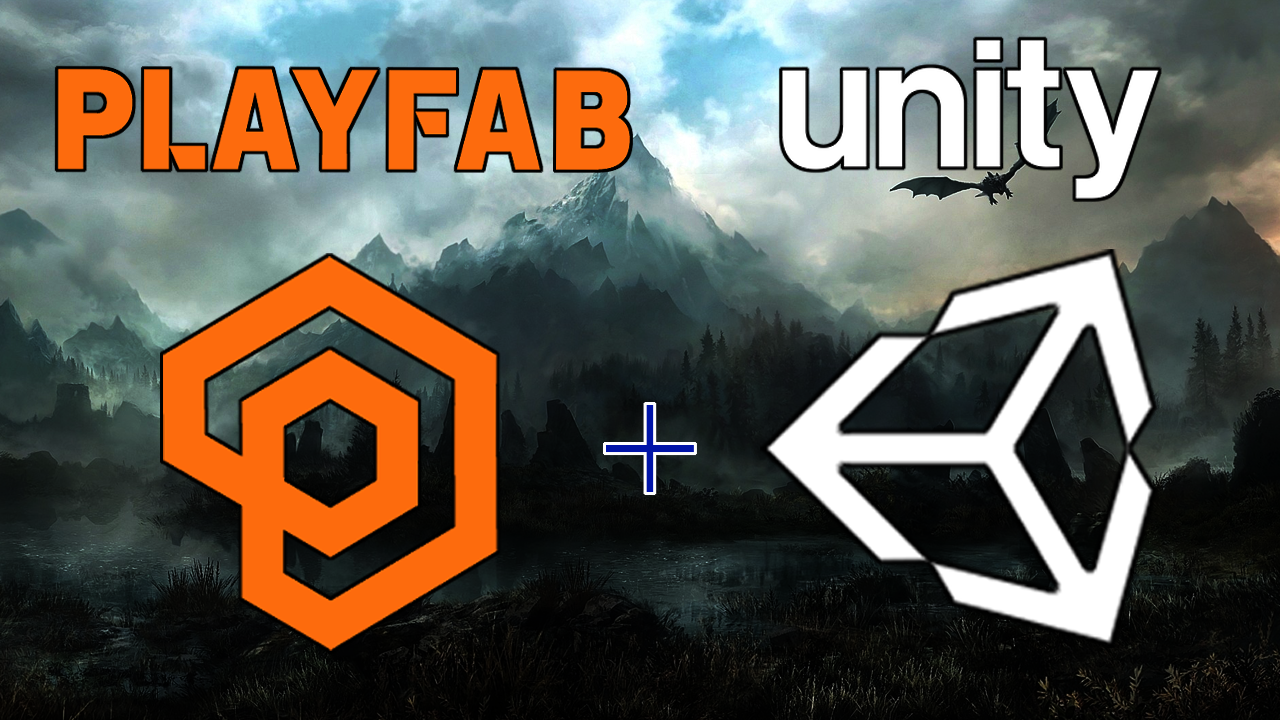
Learn to Save Data to the Cloud
For this lesson, we will teach you how to set player statistics with the PlayFab plugin. This is one of the ways to save player data to the cloud and associate the data to the player’s account.
PlayFab Doc: https://api.playfab.com/docs/tutorials/landing-players/player-statistics
Hey, welcome back to Info Gamer. We will be continuing on with part 4 of our PlayFab tutorial series. In the last lesson, we talked about Authenticating players on different platforms like Android and iOS. In this video, we will teach you how to save player data and statistics to the Playfab cloud.
Tutorial Setup
This first thing that we will do in this lesson has changed the name of our PlayFabLogin script and class. We will change it to PlayFabController. Next, we will organize our script by putting all of the functions related to the player’s login into a region that we can then collapse.
Now, before we get into the code I need to mention that the PlayFab plugin is all built upon what is called HTTP POST requests and JSON files to send and save player data to the cloud. If you are familiar with networking or web development then you should know all about HTTP Post requests but if you are not just know that it is a very common method used to send data across the internet.
Creating Variables
Now that you know more about using the PlayFab SDK we can start programming. First, we will create some variables to hold the different statistics of the player. These variables need to be 32-bit integers and can be any variable that you might need to represent the player’s stats or even the state of the game.
Once you have some variables created you will then need to make it so you can access these variables from anywhere in your game. The easiest way to do this is to create a singleton of your PlayFabController script.
Get and Set Player Statistics
Next, we will need to create a new function for setting the player’s statistics The documentation link to above provides an example of this function. You will want to customize this function so that it sends all of you stat variables in the Update list. We will then create a Get Stats function. You can copy the example functions from the documentation. You will then need to customize the callback function “OnGetStats(),” so that it then sets your state variables with the resulting values. In this step, it is important to use a switch statement to set the correct values.
You will then need to call your GetStat function in all of your call back function from successfully logging in the players. After this, you can save your script.
Before we play the game you will need to go to the PlayFab dashboard of our game. We need to enable client requests to set statistical data. Follow these steps:
– Log into PlayFab
– Click your title
– Select “Settings” from the left-menu
– Select the “API Features” tab
– Find and activate “Allow client to post player statistics”
But please note that doing so disables a security layer for your title, allowing players to post arbitrary scores to all of their statistics. If your game has any competitive play aspect, we would recommend that you never post statistics from the client.
Working in Unity
Once back in Unity you will want to create a new UI button to pair the SetStat function which needs to be a public function. You will do this by adding an OnClick event, drag in the PlayFabController object from your hierarchy. Then you the drop-down menu to select the SetStats function.
Testing your Project
Now you can play your game. After login in, you can select your PlayFabController game object and in the inspector, you can manually set the stats variables. You can then click on your newly created button. this should then send a POST request to the PlayFab cloud where the data will be saved. Now if you restart your game you will notice that the stats variable will automatically be populated with the same value shortly after logging into your PlayFab account.
I hope you enjoyed this lesson and were able to follow along. If you need help, leave your question in the comments below or join our discord. Make sure you subscribe to our channel so you can be up to date on all our latest videos.
Watch How to Cloud Save Player Data
PlayFabController.cs
using PlayFab; using PlayFab.ClientModels; using UnityEngine; using PlayFab.DataModels; using PlayFab.ProfilesModels; using System.Collections.Generic; public class PlayFabController : MonoBehaviour { public static PlayFabController PFC; private string userEmail; private string userPassword; private string username; public GameObject loginPanel; public GameObject addLoginPanel; public GameObject recoverButton; private void OnEnable() { if(PlayFabController.PFC == null) { PlayFabController.PFC = this; } else { if(PlayFabController.PFC != this) { Destroy(this.gameObject); } } DontDestroyOnLoad(this.gameObject); } public void Start() { //Note: Setting title Id here can be skipped if you have set the value in Editor Extensions already. if (string.IsNullOrEmpty(PlayFabSettings.TitleId)) { PlayFabSettings.TitleId = "8741"; // Please change this value to your own titleId from PlayFab Game Manager } //PlayerPrefs.DeleteAll(); //var request = new LoginWithCustomIDRequest { CustomId = "GettingStartedGuide", CreateAccount = true }; //PlayFabClientAPI.LoginWithCustomID(request, OnLoginSuccess, OnLoginFailure); if (PlayerPrefs.HasKey("EMAIL")) { userEmail = PlayerPrefs.GetString("EMAIL"); userPassword = PlayerPrefs.GetString("PASSWORD"); var request = new LoginWithEmailAddressRequest { Email = userEmail, Password = userPassword }; PlayFabClientAPI.LoginWithEmailAddress(request, OnLoginSuccess, OnLoginFailure); } else { #if UNITY_ANDROID var requestAndroid = new LoginWithAndroidDeviceIDRequest { AndroidDeviceId = ReturnMobileID(), CreateAccount = true }; PlayFabClientAPI.LoginWithAndroidDeviceID(requestAndroid, OnLoginMobileSuccess, OnLoginMobileFailure); #endif #if UNITY_IOS var requestIOS = new LoginWithIOSDeviceIDRequest { DeviceId = ReturnMobileID(), CreateAccount = true }; PlayFabClientAPI.LoginWithIOSDeviceID(requestIOS, OnLoginMobileSuccess, OnLoginMobileFailure); #endif } } #region Login private void OnLoginSuccess(LoginResult result) { Debug.Log("Congratulations, you made your first successful API call!"); PlayerPrefs.SetString("EMAIL", userEmail); PlayerPrefs.SetString("PASSWORD", userPassword); loginPanel.SetActive(false); recoverButton.SetActive(false); GetStats(); } private void OnLoginMobileSuccess(LoginResult result) { Debug.Log("Congratulations, you made your first successful API call!"); GetStats(); loginPanel.SetActive(false); } private void OnRegisterSuccess(RegisterPlayFabUserResult result) { Debug.Log("Congratulations, you made your first successful API call!"); PlayerPrefs.SetString("EMAIL", userEmail); PlayerPrefs.SetString("PASSWORD", userPassword); GetStats(); loginPanel.SetActive(false); } private void OnLoginFailure(PlayFabError error) { var registerRequest = new RegisterPlayFabUserRequest { Email = userEmail, Password = userPassword, Username = username }; PlayFabClientAPI.RegisterPlayFabUser(registerRequest, OnRegisterSuccess, OnRegisterFailure); } private void OnLoginMobileFailure(PlayFabError error) { Debug.Log(error.GenerateErrorReport()); } private void OnRegisterFailure(PlayFabError error) { Debug.LogError(error.GenerateErrorReport()); } public void GetUserEmail(string emailIn) { userEmail = emailIn; } public void GetUserPassword(string passwordIn) { userPassword = passwordIn; } public void GetUsername(string usernameIn) { username = usernameIn; } public void OnClickLogin() { var request = new LoginWithEmailAddressRequest { Email = userEmail, Password = userPassword }; PlayFabClientAPI.LoginWithEmailAddress(request, OnLoginSuccess, OnLoginFailure); } public static string ReturnMobileID() { string deviceID = SystemInfo.deviceUniqueIdentifier; return deviceID; } public void OpenAddLogin() { addLoginPanel.SetActive(true); } public void OnClickAddLogin() { var addLoginRequest = new AddUsernamePasswordRequest { Email = userEmail, Password = userPassword, Username = username }; PlayFabClientAPI.AddUsernamePassword(addLoginRequest, OnAddLoginSuccess, OnRegisterFailure); } private void OnAddLoginSuccess(AddUsernamePasswordResult result) { Debug.Log("Congratulations, you made your first successful API call!"); GetStats(); PlayerPrefs.SetString("EMAIL", userEmail); PlayerPrefs.SetString("PASSWORD", userPassword); addLoginPanel.SetActive(false); } #endregion Login public int playerLevel; public int gameLevel; public int playerHealth; public int playerDamage; public int playerHighScore; #region PlayerStats public void SetStats() { PlayFabClientAPI.UpdatePlayerStatistics(new UpdatePlayerStatisticsRequest { // request.Statistics is a list, so multiple StatisticUpdate objects can be defined if required. Statistics = new List<StatisticUpdate> { new StatisticUpdate { StatisticName = "PlayerLevel", Value = playerLevel }, new StatisticUpdate { StatisticName = "GameLevel", Value = gameLevel }, new StatisticUpdate { StatisticName = "PlayerHealth", Value = playerHealth }, new StatisticUpdate { StatisticName = "PlayerDamage", Value = playerDamage }, new StatisticUpdate { StatisticName = "PlayerHighScore", Value = playerHighScore }, } }, result => { Debug.Log("User statistics updated"); }, error => { Debug.LogError(error.GenerateErrorReport()); }); } void GetStats() { PlayFabClientAPI.GetPlayerStatistics( new GetPlayerStatisticsRequest(), OnGetStats, error => Debug.LogError(error.GenerateErrorReport()) ); } void OnGetStats(GetPlayerStatisticsResult result) { Debug.Log("Received the following Statistics:"); foreach (var eachStat in result.Statistics) { Debug.Log("Statistic (" + eachStat.StatisticName + "): " + eachStat.Value); switch(eachStat.StatisticName) { case "PlayerLevel": playerLevel = eachStat.Value; break; case "GameLevel": gameLevel = eachStat.Value; break; case "PlayerHealth": playerHealth = eachStat.Value; break; case "PlayerDamage": playerDamage = eachStat.Value; break; case "PlayerHighScore": playerHighScore = eachStat.Value; break; } } } #endregion PlayerStats }
using Photon.Chat; using Photon.Pun; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class PhotonChatManager : MonoBehaviour, IChatClientListener { #region Setup [SerializeField] GameObject joinChatButton; ChatClient chatClient; bool isConnected; [SerializeField] string username; public void UsernameOnValueChange(string valueIn) { username = valueIn; } public void ChatConnectOnClick() { isConnected = true; chatClient = new ChatClient(this); //chatClient.ChatRegion = "US"; chatClient.Connect(PhotonNetwork.PhotonServerSettings.AppSettings.AppIdChat, PhotonNetwork.AppVersion, new AuthenticationValues(username)); Debug.Log("Connenting"); } #endregion Setup #region General [SerializeField] GameObject chatPanel; string privateReceiver = ""; string currentChat; [SerializeField] InputField chatField; [SerializeField] Text chatDisplay; // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { if (isConnected) { chatClient.Service(); } if (chatField.text != "" && Input.GetKey(KeyCode.Return)) { SubmitPublicChatOnClick(); SubmitPrivateChatOnClick(); } } #endregion General #region PublicChat public void SubmitPublicChatOnClick() { if (privateReceiver == "") { chatClient.PublishMessage("RegionChannel", currentChat); chatField.text = ""; currentChat = ""; } } public void TypeChatOnValueChange(string valueIn) { currentChat = valueIn; } #endregion PublicChat #region PrivateChat public void ReceiverOnValueChange(string valueIn) { privateReceiver = valueIn; } public void SubmitPrivateChatOnClick() { if (privateReceiver != "") { chatClient.SendPrivateMessage(privateReceiver, currentChat); chatField.text = ""; currentChat = ""; } } #endregion PrivateChat #region Callbacks public void DebugReturn(DebugLevel level, string message) { //throw new System.NotImplementedException(); } public void OnChatStateChange(ChatState state) { if(state == ChatState.Uninitialized) { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } } public void OnConnected() { Debug.Log("Connected"); joinChatButton.SetActive(false); chatClient.Subscribe(new string[] { "RegionChannel" }); } public void OnDisconnected() { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } public void OnGetMessages(string channelName, string[] senders, object[] messages) { string msgs = ""; for (int i = 0; i < senders.Length; i++) { msgs = string.Format("{0}: {1}", senders[i], messages[i]); chatDisplay.text += "\n" + msgs; Debug.Log(msgs); } } public void OnPrivateMessage(string sender, object message, string channelName) { string msgs = ""; msgs = string.Format("(Private) {0}: {1}", sender, message); chatDisplay.text += "\n " + msgs; Debug.Log(msgs); } public void OnStatusUpdate(string user, int status, bool gotMessage, object message) { throw new System.NotImplementedException(); } public void OnSubscribed(string[] channels, bool[] results) { chatPanel.SetActive(true); } public void OnUnsubscribed(string[] channels) { throw new System.NotImplementedException(); } public void OnUserSubscribed(string channel, string user) { throw new System.NotImplementedException(); } public void OnUserUnsubscribed(string channel, string user) { throw new System.NotImplementedException(); } #endregion Callbacks }