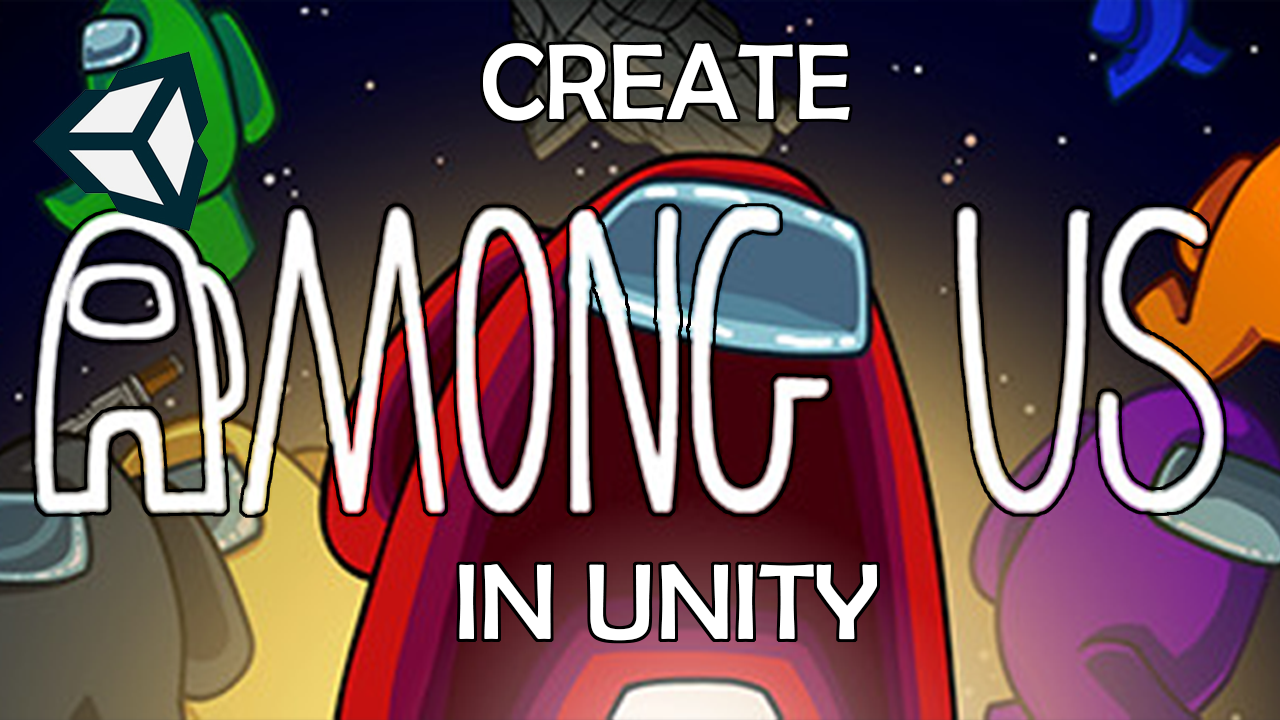
In this lesson on how to make Among Us in Unity, we will show you how to create a simple character customization menu for changing the player’s color. We will begin by creating the menu and the menu is made up of a few panels and a button for each color.
We will then create a the character customizer script. This script will just have one function that is used for each button in our menu. This function will take a color and send it to the player so the player can use that color to set its sprite color.
AU_CharacterCustomizer.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.SceneManagement; public class AU_CharacterCustomizer : MonoBehaviour { [SerializeField] Color[] allColors; public void SetColor(int colorIndex) { AU_PlayerController.localPlayer.SetColor(allColors[colorIndex]); } public void NextScene(int sceneIndex) { SceneManager.LoadScene(sceneIndex); } }
AU_PlayerController.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.InputSystem; public class AU_PlayerController : MonoBehaviour { [SerializeField] bool hasControl; public static AU_PlayerController localPlayer; //Components Rigidbody myRB; Animator myAnim; Transform myAvatar; //Player movement [SerializeField] InputAction WASD; Vector2 movementInput; [SerializeField] float movementSpeed; //Player Color static Color myColor; SpriteRenderer myAvatarSprite; private void OnEnable() { WASD.Enable(); } private void OnDisable() { WASD.Disable(); } // Start is called before the first frame update void Start() { if(hasControl) { localPlayer = this; } myRB = GetComponent<Rigidbody>(); myAnim = GetComponent<Animator>(); myAvatar = transform.GetChild(0); myAvatarSprite = myAvatar.GetComponent<SpriteRenderer>(); if (myColor == Color.clear) myColor = Color.white; myAvatarSprite.color = myColor; } // Update is called once per frame void Update() { movementInput = WASD.ReadValue<Vector2>(); myAnim.SetFloat("Speed", movementInput.magnitude); if (movementInput.x != 0) { myAvatar.localScale = new Vector2(Mathf.Sign(movementInput.x), 1); } } private void FixedUpdate() { myRB.velocity = movementInput * movementSpeed; } public void SetColor(Color newColor) { myColor = newColor; if (myAvatarSprite != null) { myAvatarSprite.color = myColor; } } }
using Photon.Chat; using Photon.Pun; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class PhotonChatManager : MonoBehaviour, IChatClientListener { #region Setup [SerializeField] GameObject joinChatButton; ChatClient chatClient; bool isConnected; [SerializeField] string username; public void UsernameOnValueChange(string valueIn) { username = valueIn; } public void ChatConnectOnClick() { isConnected = true; chatClient = new ChatClient(this); //chatClient.ChatRegion = "US"; chatClient.Connect(PhotonNetwork.PhotonServerSettings.AppSettings.AppIdChat, PhotonNetwork.AppVersion, new AuthenticationValues(username)); Debug.Log("Connenting"); } #endregion Setup #region General [SerializeField] GameObject chatPanel; string privateReceiver = ""; string currentChat; [SerializeField] InputField chatField; [SerializeField] Text chatDisplay; // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { if (isConnected) { chatClient.Service(); } if (chatField.text != "" && Input.GetKey(KeyCode.Return)) { SubmitPublicChatOnClick(); SubmitPrivateChatOnClick(); } } #endregion General #region PublicChat public void SubmitPublicChatOnClick() { if (privateReceiver == "") { chatClient.PublishMessage("RegionChannel", currentChat); chatField.text = ""; currentChat = ""; } } public void TypeChatOnValueChange(string valueIn) { currentChat = valueIn; } #endregion PublicChat #region PrivateChat public void ReceiverOnValueChange(string valueIn) { privateReceiver = valueIn; } public void SubmitPrivateChatOnClick() { if (privateReceiver != "") { chatClient.SendPrivateMessage(privateReceiver, currentChat); chatField.text = ""; currentChat = ""; } } #endregion PrivateChat #region Callbacks public void DebugReturn(DebugLevel level, string message) { //throw new System.NotImplementedException(); } public void OnChatStateChange(ChatState state) { if(state == ChatState.Uninitialized) { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } } public void OnConnected() { Debug.Log("Connected"); joinChatButton.SetActive(false); chatClient.Subscribe(new string[] { "RegionChannel" }); } public void OnDisconnected() { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } public void OnGetMessages(string channelName, string[] senders, object[] messages) { string msgs = ""; for (int i = 0; i < senders.Length; i++) { msgs = string.Format("{0}: {1}", senders[i], messages[i]); chatDisplay.text += "\n" + msgs; Debug.Log(msgs); } } public void OnPrivateMessage(string sender, object message, string channelName) { string msgs = ""; msgs = string.Format("(Private) {0}: {1}", sender, message); chatDisplay.text += "\n " + msgs; Debug.Log(msgs); } public void OnStatusUpdate(string user, int status, bool gotMessage, object message) { throw new System.NotImplementedException(); } public void OnSubscribed(string[] channels, bool[] results) { chatPanel.SetActive(true); } public void OnUnsubscribed(string[] channels) { throw new System.NotImplementedException(); } public void OnUserSubscribed(string channel, string user) { throw new System.NotImplementedException(); } public void OnUserUnsubscribed(string channel, string user) { throw new System.NotImplementedException(); } #endregion Callbacks }